What is a Vector in C++
Vector in C++ is a type of STL container which is used for storing elements in sequential manner just like arrays. One major difference between Vectors and arrays is that in case of array the size is fixed. Changing size of an array dynamically is not at all feasible. However with Vectors, we are having advantage of changing size dynamically based on need.
For Example
int a[5]; // Array a with fixed size 5
int b[100]; // Array b with fixed size 100
char c[300]; // Array c with fixedsize 300
As seen above, we have created array a, b and c of size 5,100 and 300. There is no provision to change the size later on. Therefore, if we try to access location a[20] or b[300] it shall generate runtime crash .
However, with vector we do not have such limitations. We can say vector is a more dynamic form of arrays where it is having all its feature of insertion, deletion plus change of size also.
When to implement Vector
Below are the ideal cases for implementing or using a Vector inside an application:
- Not aware how many elements we can store at runtime
- Need sequential access of elements in fixed time
- Mainly a new element should get inserted at the end position
In conclusion, if above all statements above are true, then we have ideal case to implement Vector in your application.
Which Header File for Vector
We need to include below header file in our application if we want to implement Vector in our application.
#include<vector>
How to create Vectors
Like array we can create Vector for any data type, including user-defined data types also.
For example
vector<int> i; // vector i for integer data
vector<char> c; // vector c for character data
vector<float> f; // vector f for float data
vector<a> ud; // vector ud for user-defined data type "a"
How to Initialize a Vector
Like array, we can also initialize Vector.
For example:
vector<int> i ={1,2,3,4,5}; // vector i initialized vector<char> c ={'a','b'}; // vector c init. with a and b
How to print size of a Vector
In below section, details about Vector operators for inserting and printing elements are captured.
size( ) : Printing Current Size of vector
In order to print current size of Vector we are having size( ) method.
Below example is depicting how to get the current size of Vectors.
#include <iostream>
#include <vector>
using namespace std;
int main ()
{
vector<int> v; // vector v for storing int
cout << "size: " << v.size() << endl;
// printing size of vector
return 0;
}
Output

As seen above, we have created Vector v for storing integer elements. At anytime, we can print the size of vector using size( ). For above example, we have not added any element therefore we shall get size as 0.
How to print Vector elements
iterator: for iterating element of vector.
For iterating or accessing element in array we need index location and with that we can access its element.
For example
int a[5]; //Array of integer
for(int i=0; i<5; i++)
cout<< a[i] << endl;
Similarly, in Vector for accessing its element we have iterator. Iterator support us for accessing all elements of a Vector.
For example, below application depicting how to implement iterator for Vector:
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
vector<int> v ={1,2,3,4,5};
vector<int>::iterator it;
cout<<"Printing vector elements"<<endl;
for(it=v.begin();it!= v.end(); ++it)
{
cout<< *it << endl;
}
return 0;
}
vector: begin( ) and end( ) for returning front and end elements
array[0] return the first element and array[n-1] return the last element of the array. Similarly we can get first and last element for Vector with begin( ) and end( ) members.
vector<int> v; v.begin() // return iterator at front position v.end() // return iterator at last position
How to insert a new element in Vectors
push_back( ): Inserting element at last
Since vector is a sequential container, therefore, at any time we can insert element at its end position by using push_back( ).
For example, in order to add 3 elements in vector we need to call push_back( ) 3 times. Below is the example for adding 3 elements at the end of a Vector.
#include <iostream>
#include <vector>
using namespace std;
int main ()
{
vector<int> v;
cout << "Initial size: " << v.size() << endl;
v.push_back(10);
v.push_back(20);
v.push_back(30);
vector<int>::iterator it;
cout << "After pushing 3 elements size: " << v.size() << endl;
for(it = v.begin(); it != v.end(); ++it)
{
cout<<*it<<endl;
}
cout << "Final size: " << v.size() << endl;
return 0;
}
In above example, after calling push_back( ) 3 times, the size of vector got changed to 3 from 0.
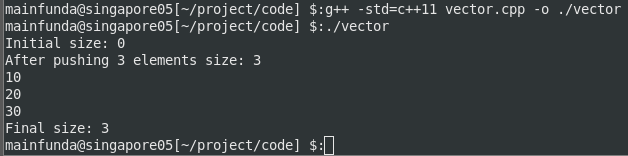
In conclusion, we are dynamically changing the size of vector by adding element at runtime.
insert( ): Inserting an element at any specific position
In order to add element at any position in Vector, we are having insert( ) member.
For example, below is the sample application for inserting an element at any location:
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
vector<int> v ={1,2,3};
cout << "Initial size: " << v.size() << endl;
v.insert(v.begin(), 40);
cout << "After Inserting at beginning: " << v.size() << endl;
v.insert(v.begin()+4,50);
cout << "After inserting at 4th position: " << v.size() << endl;
vector<int>::iterator it;
cout << "The vector contents:" << endl;
for(it = v.begin(); it != v.end(); ++it)
{
cout<<*it<<endl;
}
return 0;
}
As seen in above application, we have inserted data at the beginning and at 4th position of Vector v.
emplace( ): For inserting data
We can also insert data at any position by using emplace( ) operator . It will increase the size of vector by 1.
The detailed analysis between push & emplace is present in C++ vector : emplace_back Vs push_back
Below is the example for the same:
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
std::vector<int> v = {1,2,3};
cout<<"vector size "<<v.size()<<endl;
v.emplace ( v.begin(), 5 );
v.emplace ( v.end(), 8 );
cout<<"After emplace vector size "<<v.size()<<endl;
return 0;
}
Output

How to delete element from Vector
In array, there is no option to shrink the size of array or remove an element. However, in Vector we have support for erasing an element or clearing all its elements.
erase( ) : erasing or deleting element from vector
For example, below sample application is erasing 2nd element from vector.
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
vector<int> v ={1,2,3,4,5};
cout << "Initial size: " << v.size() << endl;
vector<int>::iterator it;
cout<<"Printing vector elements"<<endl;
for(it=v.begin();it!=v.end(); ++it)
{
cout<<*it<<endl;
}
v.erase (v.begin()+2); // remove the 3rd element
cout<<"Printing vector elements after erasing"<<endl;
for(it=v.begin();it< v.end(); ++it)
{
cout<<*it<<endl;
}
cout << "After erasing 2nd elements size: "
<< v.size() << endl;
return 0;
}
Output

clear( ): Clearing or removing all elements
Below example demonstrating how to erase or clear all elements from vector:
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
vector<int> v ={1,2,3,4,5};
cout << "Initial size: " << v.size() << endl;
vector<int>::iterator it;
cout<<"Printing vector elements"<<endl;
for(it=v.begin();it!=v.end(); ++it)
{
cout<<*it<<endl;
}
v.clear (); // clear all element
cout<<"Printing vector elements after erasing"<<endl;
for(it=v.begin();it< v.end(); ++it)
{
cout<<*it<<endl;
}
cout << "After erasing 2nd elements size: " << v.size() << endl;
return 0;
}
Output

How to allocate Vector in C++
As seen above, size( ) returns the number of elements the vector is holding at that time. But we also have members for dealing with allocation.
capacity( ): Storage allocations
It returns the maximum storage allocated for vector at that moment. However, such allocation can be larger or equal to size of the Vector. Ideally, capacity depicts how many elements vector can accommodate without doing reallocation.
For example: Below applciation is printing current capacity and size of the Vector.
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
vector<int> v;
cout << "Initial size: " << v.size() << endl;
cout << "Initial capacity: " << v.capacity() << endl;
for(int i =0;i<1000;i++)
v.push_back(30);
cout << "After pushing elements size: "
<< v.size() << endl;
cout << "After pushing elements capacity: "
<< v.capacity() << endl;
return 0;
}
Output

max_size( ): print maximum allocation possible
In order to print maximum element which Vector can accommodate, we have max_size( ). It depicts the maximum size which container can take. There is also no guaranty that container will reach such size, it again depends on system library implementation.
Below ex:
#include <iostream> //main header for io
#include <vector> //for vectors
using namespace std; //for namespace
int main ()
{
vector<int> v;
cout << "Initial size: " << v.size() << endl;
cout << "Initial capacity: " << v.capacity() << endl;
cout<< "Maximum allocation : "<<v.max_size()<<endl;
for(int i =0;i<1000;i++)
v.push_back(30);
cout << "After pushing elements size: "
<< v.size() << endl;
cout << "After pushing elements capacity: "
<< v.capacity() << endl;
cout << "After pushing elements capacity: "
<< v.max_size() << endl;
return 0;
}
Output

How Vector in C++ do Memory management
As we know, Vector supports dynamic memory allocation. So, it is possible to keep inserting element in vector. But it may happen that next memory allocation is not possible and in this case, vector needs to reallocate all elements and move them to new larger allocation. Such process seems to be an overhead for system performance. Therefore, in order to save such reallocation, the vector always allocates more memory than is required. Therefore, Vectors are having 2 operations, first is size( ) which gives current memory required for storing all elements. And second is the capacity( ), which gives how much elements vector can insert without reallocation.
Is Vectors Thread safe?
Vector operations are not thread safe. If you are inserting element in one thread and deleting element in other thread, then there is need to protect the vector using thread synchronization mechanism.
Vector: Comparison with Arrays
Feature | Vector | Array |
Sequential | Yes | Yes |
Accessing element with index in O(1). | Yes | Yes |
Dynamic size change | Yes | No |
Memory need | More – usually allocate more in order to manage dynamic size . | Fixed |
Insertion and deletion of element | Efficient at end. Worst – Another position. Need to reallocate all elements. | Not possible |
Thread safe | No | No |
Vector: Time requirement
Feature | Vector time |
Deletion at end | Constant time -O(1) |
Insertion at end | Differential time – O(kn) Sometime needs to extend vector |
Insertion and deletion at any other point | Linear time- O(n) |
size | Linear time-O(n) |
find | Linear time-O(n) |
Main Funda: Vector in C++ is substitute of traditional array with much better usability.